Introduction
Detailed walkthroughs for Chrome CTF challenges on TryHackMe .
Description
A password manager is only as strong as the password that encrypts it. You find that a malicious actor extracted something over the network, but what? Help us find out!
1.What is the first password that we find?
2.What is the URL found in the first index? Fully defang the URL
3.What is the password found in the first index?
4.What is the URL found in the second index? Fully defang the URL
5.What is the password found in the second index?
From the above questions, we assume that will find the paswords and urls using the task file.
Enumaration
We downloaded the task file from Chrome|THM
page, and unzipped it. We got a .pcapng
file.
-rw-r--r-- 1 root root 73M Mar 18 21:36 chromefiles-1697949492677.zip
-rwxr-xr-x 1 root root 73M Oct 22 2023 traffic.pcapng
Wireshark
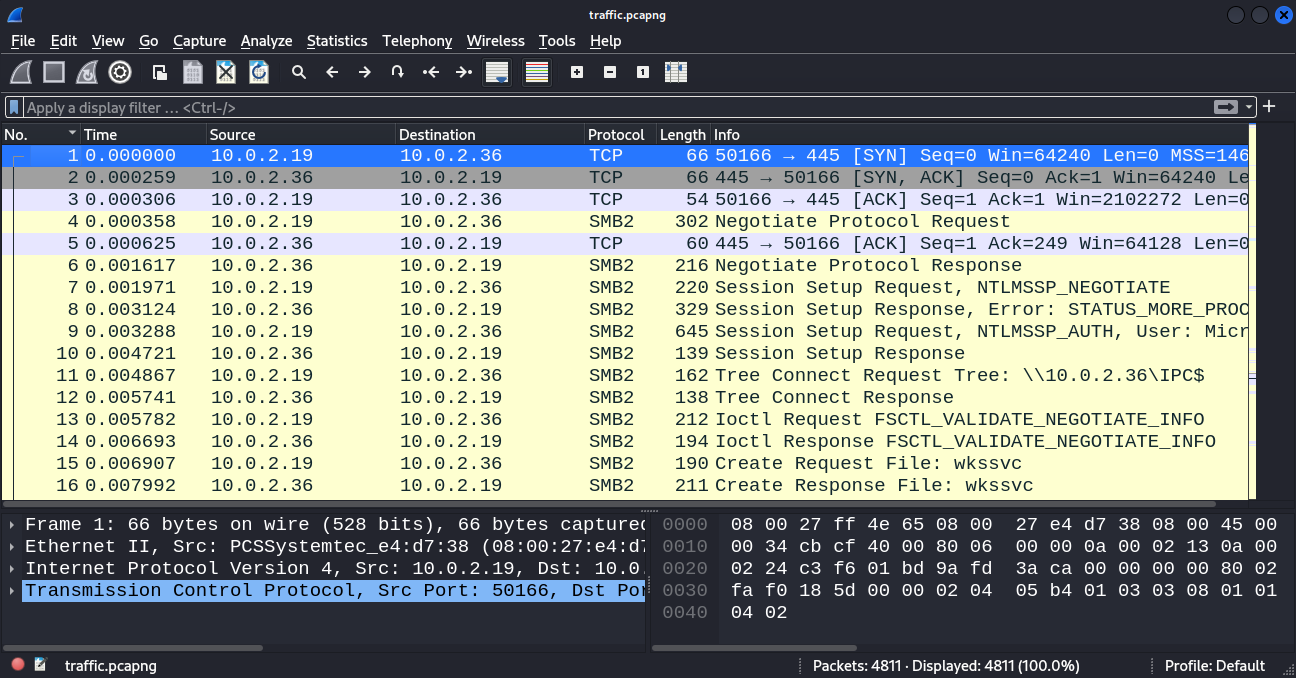
Statics --> Protocol Hieraracy
, we have SMB
data in the captured file.
-rw-r--r-- 1 root root 72M Aug 18 22:33 encrypted_files
-rw-r--r-- 1 root root 160 Aug 18 22:36 srvsvc
-rw-r--r-- 1 root root 5.5K Aug 18 22:36 transfer.exe
ILSpy
Lets try to get more information from these files, start with transfer.exe
. Here we need to reverse .exe
file, using git/icsharpcode/ilspy-vscode
reference –>HackTricks:ReversingTools:ILSpy
–> ILSpy Extension
.
ILSpy Extension VSCode
extension.
Decompile Selected assembly
to decompile the file.
- Reads the data from
C:\Users\hadri\Downloads\files.zip
and encrypt asC:\Users\hadri\Downloads\encrypted_files
. - Encryption algorithm used is
AES
. - Key:
PjoM95MpBdz85Kk7ewcXSLWCoAr7mRj1
- IV (Initialization Vector):
lR3soZqkaWZ9ojTX
Decrypting AES
Lets decrypt the encrypted file.
from Crypto.Cipher import AES
from Crypto.Util.Padding import unpad
import sys
# Replace these with your actual decryption key and initialization vector (IV)
key = b"PjoM95MpBdz85Kk7ewcXSLWCoAr7mRj1" # 16-byte key (128-bit)
iv = b"lR3soZqkaWZ9ojTX" # 16-byte IV
def decrypt_file(encrypted_file_path, decrypted_file_path):
"""Decrypts a file using AES-128 in CBC mode with PKCS#7 padding.
Args:
encrypted_file_path (str): Path to the encrypted file.
decrypted_file_path (str): Path to save the decrypted file.
"""
with open(encrypted_file_path, 'rb') as encrypted_file:
ciphertext = encrypted_file.read()
cipher = AES.new(key, AES.MODE_CBC, iv)
plaintext = unpad(cipher.decrypt(ciphertext), AES.block_size)
with open(decrypted_file_path, 'wb') as decrypted_file:
decrypted_file.write(plaintext)
# Example usage (replace paths accordingly)
encrypted_file_path = sys.argv[1]
decrypted_file_path = sys.argv[2]
decrypt_file(encrypted_file_path, decrypted_file_path)
print("File decrypted successfully!")
I fed the transfer.exe contents, containing an encryption mechanism, to an AI tool, and it produced the necessary decryption script. Save the above python script as decryption.py
.
pip3 install pycryptodome # to use Crypto in Python env
python3 decryption.py encrypted_files decrypted_file.zip
unzip decrypted_file.zip -d decrypted_files
Now we got the decrypted contents of the file encrypted_files. Let’s go through the files.
AppData [Windows]
We have the AppData
file of Windows OS, which is a hidden system folder that stores application data and settings specific to the user profile. It is used by Windows and installed applications to store user-specific information such as configuration files, temporary files, saved sessions, caches, and other necessary data.
Solving using Linux
$ file decrypted_files/AppData/Local/Google/Chrome/User\ Data/Default/Login\ Data
decrypted_files/AppData/Local/Google/Chrome/User Data/Default/Login Data: SQLite 3.x database, last written using SQLite version 3039004, page size 2048, file counter 1, database pages 23, cookie 0xd, schema 4, UTF-8, version-valid-for 1
Found an interesting database file.
AppData/Roaming/Microsoft/Protect/S-1-5-21-3854677062-280096443-3674533662-1001/8c6b6187-8eaa-48bd-be16-98212a441580
AppData/Local/Google/Chrome/User\ Data/Default/Login\ Data
AppData/Local/Google/Chrome/User\ Data/Local\ State
After further enumaration found some other important files.
DB Browser for SQLite
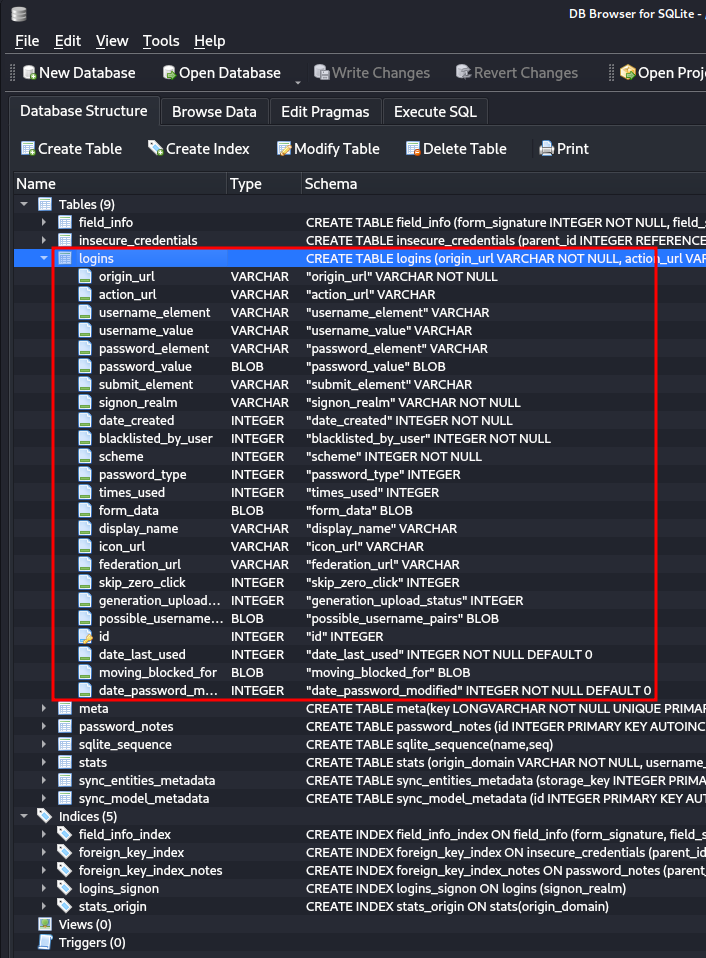
Using pre installed DB Browser for SQLite
in Kali Linux, We found an Database Structure
and interesting table logins
using the file Login Data
.
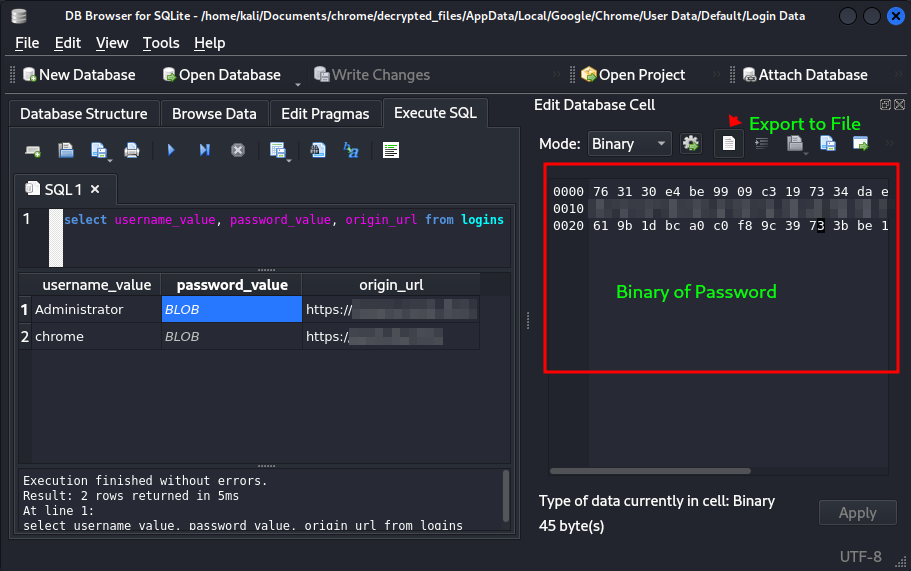
We got the important data like usernames, passwords and website urls
from the database. But here the password field contains the binary data which is unreadable.
Here we got flag2 and flag4.
DPAPImk2john
We can use DPAPImk2john
to crack the users password to proceed further.
pip install pycryptodome
# installing dependency for DPAPImk2john
$ DPAPImk2john -h
usage: DPAPImk2john [-h] [-S SID] [-mk MASTERKEY] [-d] [-c {domain,domain1607+,domain1607-,local}] [-P PREFERRED] [--password PASSWORD]
options:
-h, --help show this help message and exit
-S SID, --sid SID SID of account owning the masterkey file.
-mk MASTERKEY, --masterkey MASTERKEY
masterkey file (usually in %APPDATA%\Protect\<SID>).
-d, --debug
-c {domain,domain1607+,domain1607-,local}, --context {domain,domain1607+,domain1607-,local}
context of user account. 1607 refers to Windows 10 1607 update.
-P PREFERRED, --preferred PREFERRED
'Preferred' file containing GUID of masterkey file in use (usually in %APPDATA%\Protect\<SID>). Cannot be used with any other
command.
--password PASSWORD password to decrypt masterkey file.
DPAPImk2john Tool Usage.
$ DPAPImk2john -S "S-1-5-21-3854677062-280096443-3674533662-1001" -mk AppData/Roaming/Microsoft/Protect/S-1-5-21-3854677062-280096443-3674533662-1001/8c6b6187-8eaa-48bd-be16-98212a441580 -c "local"
$DPAPImk$2*1*S-1-5-21-3854677062-280096443-3674533662-1001*aes256*sha512*8000*46bc22dbffb7cfe4cfc5895acf364a94*288*a332e98ce791b7416157137e7193596d4594dc599acc321f6ed9e94a818879461639b958099ab61361c7fd4d6668ca2d8995488ffba78758b945dcbfc60498c84b14c8021fbee8d90cf4165637222c8a6cd1eb3c5a85acf85177b1ec86240c7e077eb5e427e27832f3481664baf54c06cb3bb47bcfc253620958f02f69bc98ece8e51b6ef3c498047efb4a2b57bf804b
$
$ DPAPImk2john -S "S-1-5-21-3854677062-280096443-3674533662-1001" -mk AppData/Roaming/Microsoft/Protect/S-1-5-21-3854677062-280096443-3674533662-1001/8c6b6187-8eaa-48bd-be16-98212a441580 -c "local" > ../DPAPIdecryption.txt
Generating the hash to decrypt using DPAPImk2john
.
$ john ../DPAPIdecryption.txt --wordlist=/usr/share/wordlists/rockyou.txt
Using default input encoding: UTF-8
Loaded 1 password hash (DPAPImk, DPAPI masterkey file v1 and v2 [SHA1/MD4 PBKDF2-(SHA1/SHA512)-DPAPI-variant 3DES/AES256 256/256 AVX2 8x])
[password-redacted] (?)
Session completed.
Cracking the passsword of the user using John the Ripper
tool.
Here we got the flag 1.
pypykatz
Here we found a tool called pypykatz , used to crack the chrome passwords.
$ pypykatz dpapi chrome -h
usage: pypykatz dpapi chrome [-h] [--logindata LOGINDATA] [--cookies COOKIES] mkf localstate
$
$ pypykatz dpapi masterkey -h
usage: pypykatz dpapi masterkey [-h] [-o OUT_FILE] masterkeyfile prekey
$
$ pypykatz dpapi prekey password test_password
usage: pypykatz password [-h] [-o OUT_FILE] sid password
Use these commands for known the tool usage.
$ pypykatz dpapi prekey password S-1-5-21-3854677062-280096443-3674533662-1001 [password-redacted]
31a620ca577e6ad02ae701673bee51d32aa5538b
16f809ed93fff267679b64095ec2ed54f20da076
266c0db66dda55bcf2a3bf948c9176f9d0073f30
$ pypykatz dpapi masterkey -o mk1.txt 8c6b6187-8eaa-48bd-be16-98212a441580 31a620ca577e6ad02ae701673bee51d32aa5538b
$ pypykatz dpapi chrome --logindata Login\ Data mk1.txt Local\ State
file: Login Data user: Administrator pass: b'[redacted-Password]' url:
file: Login Data user: chrome pass: b'[redacted-Password]' url:
We got the passwords here (Flag 3 and Flag 5).
Solving using Windows
DPAPImk2john.py
PS Z:\> pip install --user pycryptodome
PS Z:\> notepad DPAPImk2john.py
Installing dependency for DPAPImk2john.py
and copy pasting the .py
file using notepad.
Tool: JohnTheRipper
, latest version for Windowsx64
.
PS Z:\> python .\DPAPImk2john.py -S "S-1-5-21-3854677062-280096443-3674533662-1001" -mk "Z:\AppData\Roaming\Microsoft\Protect\S-1-5-21-3854677062-280096443-3674533662-1001\8c6b6187-8eaa-48bd-be16-98212a441580" -c "local" > hash.txt
PS Z:\> john-1.9.0-jumbo-1-win64\run\john.exe hash.txt --wordlist=rockyou.txt
Error: UTF-16 BOM seen in input file.
Running john.exe
to crack the password, we got the error.
PS Z:\> $InputFile = "hash.txt" ; $OutputFile = "hash_utf8.txt"; $Text = Get-Content -Path $InputFile -Raw; $Text | Out-File -FilePath $OutputFile -Encoding utf8
Converting the data from UTF-16 to UTF-8
format to solve the error and saving it in hash_utf8.txt
.
PS Z:\> john-1.9.0-jumbo-1-win64\run\john.exe .\hash_utf8.txt --wordlist=rockyou.txt
Using default input encoding: UTF-8
Loaded 1 password hash (DPAPImk, DPAPI masterkey file v1 and v2 [SHA1/MD4 PBKDF2-(SHA1/SHA512)-DPAPI-variant 3DES/AES256 256/256 AVX2 8x])
Cost 1 (iteration count) is 8000 for all loaded hashes
Will run 2 OpenMP threads
Press 'q' or Ctrl-C to abort, almost any other key for status
[password-redacted] (?)
1g 0:00:00:00 DONE (2024-09-09 06:40) 4.807g/s 307.6p/s 307.6c/s 307.6C/s 123456..charlie
Use the "--show" option to display all of the cracked passwords reliably
Session completed
Successfully cracked the user password.
mimikatz
PS Z:\> Z:\mimikatz\x64\mimikatz.exe # starting mimi
.#####. mimikatz 2.2.0 (x64) #19041 Sep 19 2022 17:44:08
.## ^ ##. "A La Vie, A L'Amour" - (oe.eo)
## / \ ## /*** Benjamin DELPY `gentilkiwi` ( benjamin@gentilkiwi.com )
## \ / ## > https://blog.gentilkiwi.com/mimikatz
'## v ##' Vincent LE TOUX ( vincent.letoux@gmail.com )
'#####' > https://pingcastle.com / https://mysmartlogon.com ***/
Lets use Mimikatz .
MASTERKEY
mimikatz # dpapi::masterkey /in:"Z:\AppData\Roaming\Microsoft\Protect\S-1-5-21-3854677062-280096443-3674533662-1001\8c6b6187-8eaa-48bd-be16-98212a441580" /sid:S-1-5-21-3854677062-280096443-3674533662-1001 /password:[password redacted] /protected
**MASTERKEYS**
dwVersion : 00000002 - 2
szGuid : {8c6b6187-8eaa-48bd-be16-98212a441580}
dwFlags : 00000005 - 5
dwMasterKeyLen : 000000b0 - 176
dwBackupKeyLen : 00000090 - 144
dwCredHistLen : 00000014 - 20
dwDomainKeyLen : 00000000 - 0
[masterkey]
**MASTERKEY**
dwVersion : 00000002 - 2
salt : 46bc22dbffb7cfe4cfc5895acf364a94
rounds : 00001f40 - 8000
algHash : 0000800e - 32782 (CALG_SHA_512)
algCrypt : 00006610 - 26128 (CALG_AES_256)
pbKey : a332e98ce791b7416157137e7193596d4594dc599acc321f6ed9e94a818879461639b958099ab61361c7fd4d6668ca2d8995488ffba78758b945dcbfc60498c84b14c8021fbee8d90cf4165637222c8a6cd1eb3c5a85acf85177b1ec86240c7e077eb5e427e27832f3481664baf54c06cb3bb47bcfc253620958f02f69bc98ece8e51b6ef3c498047efb4a2b57bf804b
[backupkey]
**MASTERKEY**
dwVersion : 00000002 - 2
salt : 16b1972d3eb971ab727d3ad4e392c1ca
rounds : 00001f40 - 8000
algHash : 0000800e - 32782 (CALG_SHA_512)
algCrypt : 00006610 - 26128 (CALG_AES_256)
pbKey : bc71f554919b4d147bf51f53950fa13278979eef7398d84ecf500988864dc52eecbe9a0201a74dbb62f38e3b7957e5aa67297c29292cba05a46007bc04f1237304ee4b064442356be165ea54e0ed2664adeb1abbeb98bfe0f5b714f00d50da8d0e9271908b036dcb2aff44f2724f41bd
[credhist]
**CREDHIST INFO**
dwVersion : 00000003 - 3
guid : {ba7fc17c-8195-4272-afae-c9fc71e42540}
[masterkey] with volatile cache: SID:S-1-5-21-3854677062-280096443-3674533662-1001;GUID:{ba7fc17c-8195-4272-afae-c9fc71e42540};MD4:753621b31cbdb532e69fcb2e64924cfe;SHA1:f6f173c285829fccc958584ac81ae15a572fd69c;
key : ca4387eb0a71fc0eea23e27f54b9ae240379c9e82a05d6fca73ecee13ca2e0e4d98390844697d8ed10715415c56152653edf460a47b70ddb868a03ee6a3f9840
sha1: 217522c457cfe8a95da45da81d6b898080e2067d
[masterkey] with password: [password redacted] (protected user)
key : ca4387eb0a71fc0eea23e27f54b9ae240379c9e82a05d6fca73ecee13ca2e0e4d98390844697d8ed10715415c56152653edf460a47b70ddb868a03ee6a3f9840
sha1: 217522c457cfe8a95da45da81d6b898080e2067d
Here we got the Master Key
. We need encrypted key to proceed further, which we found in AppData/Local/Google/Chrome/User Data/Local State
.
Encrypted Key
mimikatz # dpapi::chrome /in:"Z:\AppData\Local\Google\Chrome\User Data\Default\Login Data" /masterkey:"ca4387eb0a71fc0eea23e27f54b9ae240379c9e82a05d6fca73ecee13ca2e0e4d98390844697d8ed10715415c56152653edf460a47b70ddb868a03ee6a3f9840" /encryptedKey:RFBBUEkBAAAA0Iyd3wEV0RGMegDAT8KX6wEAAACHYWuMqo69SL4WmCEqRBWAAAAAAAIAAAAAABBmAAAAAQAAIAAAAHPuV6P/8jN+rng8E61Z0xxi2hUf4Q4oxa5gFqSnctqdAAAAAA6AAAAAAgAAIAAAAAEF9lst8zMKmCFJ3WmD46TZY/xJF+s5Xf9mTQ2wa16ZMAAAABFU2C2V+l6K3y7ROKkA0cIaWyuXB9i7zUwBBu6mt7vM2QGZtqmjhcX6ZSWrX8JUwkAAAADgBkMLAP19Rtax5T8aKAESgwV+ABz65DOgEGwwSkkQMbWrwz7p42SzpfJUj7jcyUSTOblLRNtB8YTwhm3wCQSi
> Encrypted Key seems to be protected by DPAPI
* volatile cache: GUID:{8c6b6187-8eaa-48bd-be16-98212a441580};KeyHash:217522c457cfe8a95da45da81d6b898080e2067d;Key:available
* masterkey : ca4387eb0a71fc0eea23e27f54b9ae240379c9e82a05d6fca73ecee13ca2e0e4d98390844697d8ed10715415c56152653edf460a47b70ddb868a03ee6a3f9840
> AES Key is: 9a3094f1bfe3e19d5d039fb569d35d49ad083ac34dbcd5d9e42a506b8d4a192c
URL : https://[url redacted]/ ( https://[url redacted]/ )
Username: Administrator
* using BCrypt with AES-256-GCM
Password: [password redacted]
URL : https://[url redacted]/ ( https://[url redacted]/ )
Username: chrome
* using BCrypt with AES-256-GCM
Password: [password redacted]
We solved the lab.
Happy Hacking !!! 😎